Playing with code
I'm teaching code to a friend. Both of us are graphic designers, so we set out to explore algorithms by making procedural pictures. We're enjoying our sessions a lot. I'm helping her avoid the frustration I felt, during my self-taught journey.
Having to share the logics I learned — empirically, in a long period of time — encourages me to structure my knowledge, speak out my findings. I learn as much as her! Pair programming is wonderful.
So, how do we learn to program? Clearly, tutorials loitering around on the web don't get us far. Learning the syntax doesn't get you anywhere. You need to learn how to decompose one's system complexity.
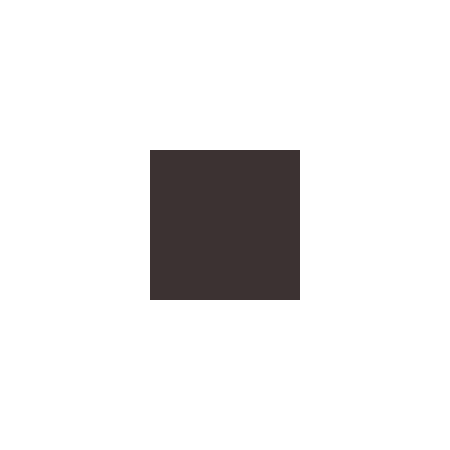
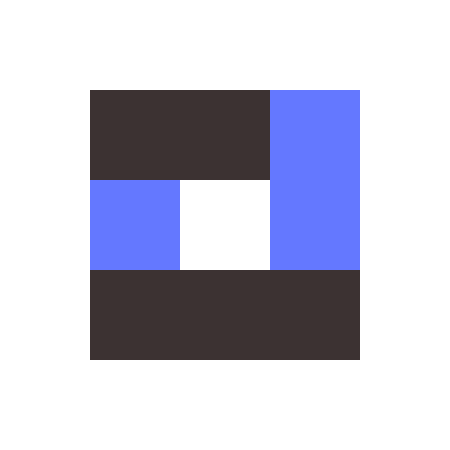
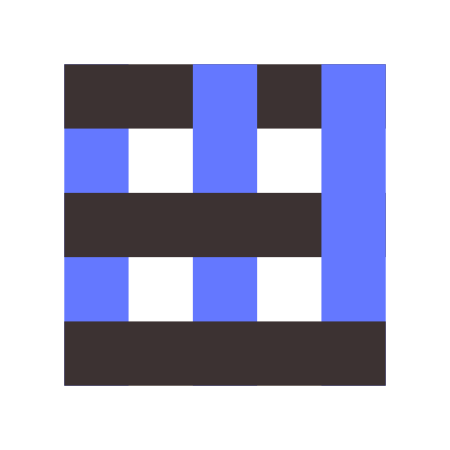
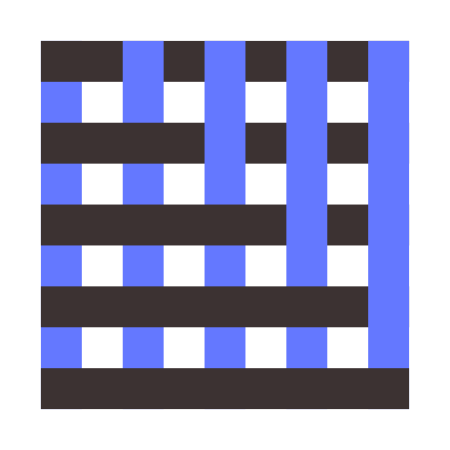
I come from the world of pictures, where the tools have been perfected for thousands of years. Comparatively, programming tools are... barbaric. It's the square of spokes in wheels. Nothing's immediate, nor visible. One must blindly manipulate symbols.
Take care of your tools; they'll take care of you.Emmanuel Corno
For us to think freely, I created an environment that requires no installation, no compilation, called Aire de Jeu. It's a blank page for programming, and the pen is just there. The difference with the classical text-file is huge. There's still much to do, of course, but it's a start. Thanks, Bret Victor, for letting me stand on your shoulders, and see further.
The game changing details:
- The tool never hangs nor crash. Stop being afraid of infinite loops!
- Numbers can be modified quickly. Drag'em like sliders!
- The API is clear, there's no hidden state, no global state, all parameters are named.
I have so many ideas. Just wait.
I'm very proud of my pupil. We just completed our fifteenth session together, & she's nearly autonomous. Let me recount our journey:
-
The bare necessities
We learned the miminum of syntax, just to play with loops:
let
,while
and the drawing functionrectangle()
, using onlynumbers
. -
Circles & Rectangles
We added another drawing function:
circle()
, and new control blocks:if
&else
. -
A loop in a loop
We discovered
scope
problems. We flexed our muscles at decomposing pictures, tried to see the repeating pattern, and recreated some of the gorgeous vintage swiss graphics. -
Abstraction
We learned to think in x. Meaning: formulate a logic that works in a general case, with a parameter. New syntax:
function()
. -
Normalize everything
We talked about percentages. 100% is not equal to
100
, it's1
. Programming teaches you good & solid mathematical foundations. -
Pi & Tau
We entered
radian
territory... Probably shouldn't have though. -
The power of naming
We learned how useful it is to express your intentions in the code. Deciding to write an equation in a form rather than in another, to bring logic to light. Taking the time to chose good
variable names
. Avoid magic numbers, create variables to use names in your equations:width/columns
rather than800/6
. -
The full team
We discovered the two remaining drawing functions:
path()
&text()
. We played with them, but rectangles & circles are funnier. -
Throw a dice
We discovered the wonders of
random()
numbers, and how to tame their power. -
Abstraction vol.2
We learned to decompose complex problems into simpler subproblems. When creating functions, we ask ourselves what would it take for these functions to be useful outside of the current example. The concept of linear interpolation revealed itself to us:
lerp(from,to,t)
. -
Abstraction vol.3
We were tired of making mistakes about loop increments & scopes, so we eased our life with a new syntax:
for
loops. It conveniently fuse all loop-related logic in one line. -
Mouse & cat
We discovered how to get
inputs
from the mouse. We played with a new, exciting kind of program: interactive pictures. It got us to think aboutvectors
. -
Mathematical creatures
We entered the forest of mathematical spirits:
sqrt()
,sinus()
,abs()
, etc.
We didn't get to know some essential blocks, and yet we succeded at crafting magnificent procedural pictures and having fun doing it. What's remaining:
- Boolean algebra:
&&
,||
,!
... - Compound structures:
[Lists]
&{objects}
Classes
,Modules
,Interfaces
, etc. All the necessities of creating programs with another order of magnitude.
Go play with this tool, and show us what you can do!
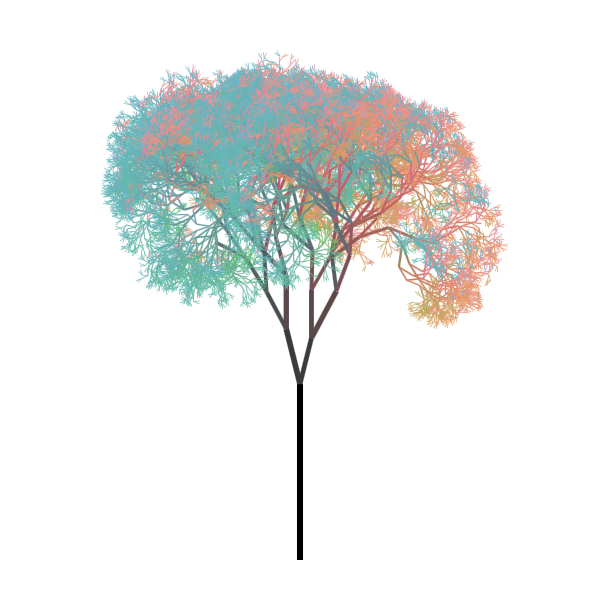
If by chance you're interested in maths, programming & pictures, I'd like to recommend some readings:
- The elements of Euclid, Oliver Byrne — Learn to see maths.
- Stop drawing dead fish, Bret Victor — What the computer is really about
- A Mathematician's Lament, Paul Lockhart — Mathematics is wonderful & easy, but school has ruined it.